Variables and the Inspector
Creating a Lua script and mounting it to a GameObject via the Para Script component is the same as attaching a custom component to this object.
Same as other Unity components, the Para Script with a Lua script can also be displayed in the [Inspector] for editing properties.
Declare Variables
If you want to declare a variable and show it in the [Inspector], you need to use the following writing rules:
- Start with ---@var , meaning to declare one variable
- Use ---@end, meaning the end of the variable declaration code block
---@var intValue:int = 0
---@var floatValue:float
---@var vectorValue:Vector3
---@var stringValues:string[]
---@var gameObject:UnityEngine.GameObject
---@var transform:UnityEngine.Transform
---@end
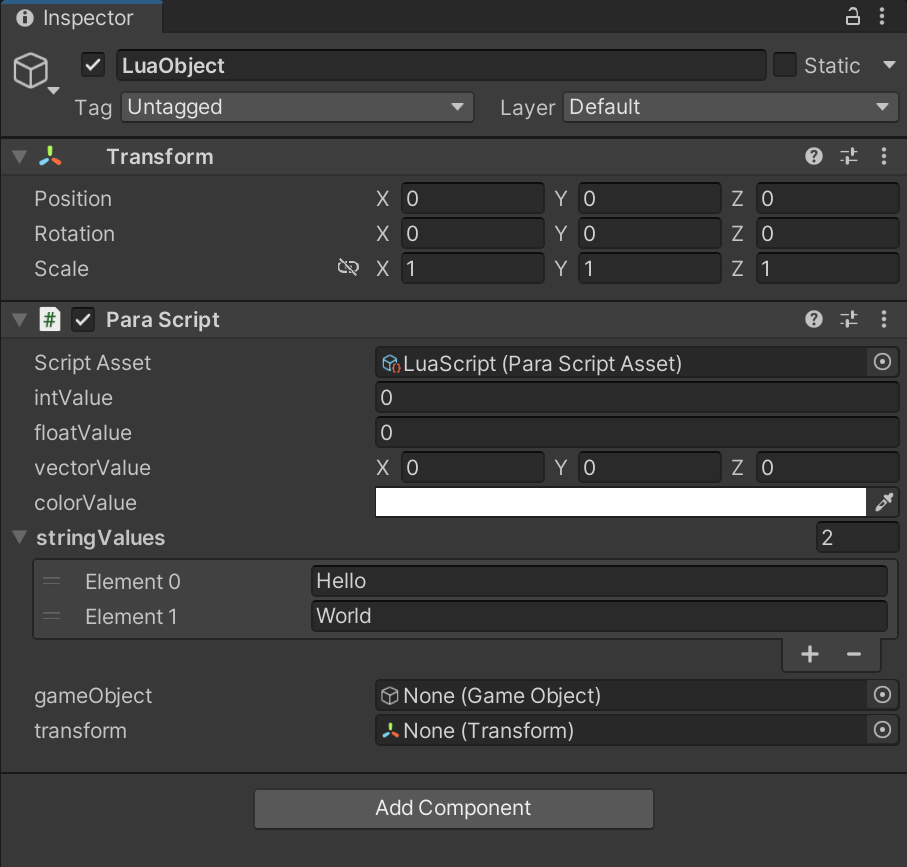
Sample Code
You can also declare and use Lua variables with Lua syntax. However, these variables will not be displayed in the [Inspector].
Further Reading: Lua Variables
Supported Variable Types
- Currently, the following variable types are supported: bool, byte, sbyte, short, ushort, int, uint, long, ulong, float, double, string, Vector2, Vector3, Vector4, Quaternion, Color
- You can also declare a variable as Unity Class and include the entire namespace when using it. For example: UnityEngine.GameObject
- You can also declare an array of any type above.
---@var intValues:int[]
---@var stringValues:string[]
---@var gameObjects:UnityEngine.GameObject[]
---@end
- You can also declare a List and Dictionary. Supported key types of Dictionary include: byte, short, int, long, string.
---@var list:List<int>
---@var dic:Dictionary<int,UnityEngine.GameObject>
Use Variables
To use a declared variable in a Lua script, just write the variable's name.
---@var message:string
---@end
-- Start is called before the first frame update
function Start()
print(message)
end
Synchronization Variable
If you want to synchronize variables between players, you can declare them as synchronization variable.
- Start with ---@varSync , meaning to declare a synchronization variable.
---@varSync intValue:int
---@varSync floatValue:float
---@varSync vectorValue:Vector3
---@end
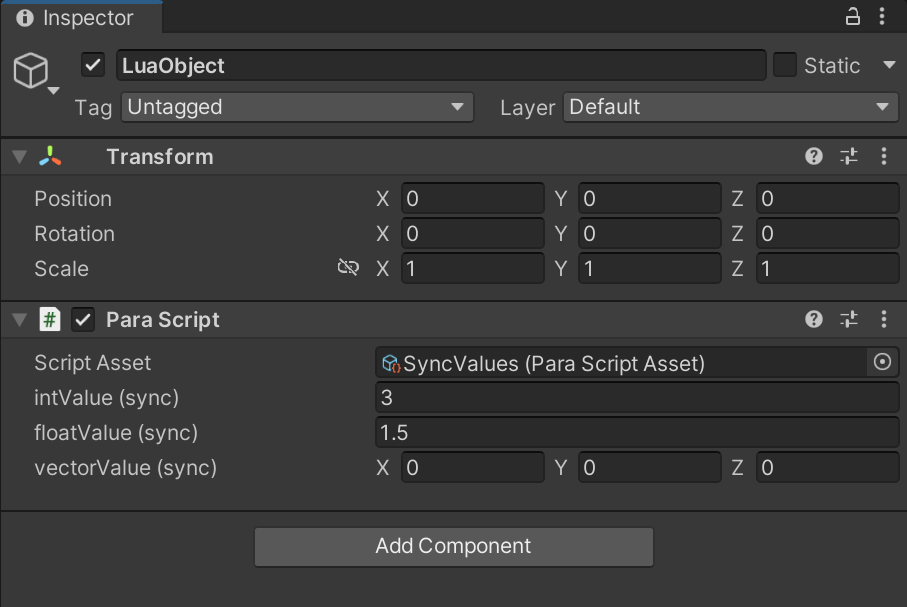
Synchronization variables are labeled with (sync).
Supported types of synchronization variable are different from those of local variable. For more details, refer to: Synced Variables.
Updated over 2 years ago