Players' Animations
You may want players in a World to play animations or modify their Animator Controller to create some interesting experiences. For example, when a player walks into a dance floor, the player will start dancing, or when a player picks up a weapon, the player will become a warrior and use some skills.
You can use the following methods to control players' animations:
API for Controlling Players' Animations | Description |
---|---|
Makes a player play an animation. | |
Makes a player stop playing a specified animation. This API is used together with PlayAnimation. | |
Overrides the current animation. | |
Overrides the current animation controller. |
Play an Animation
The PlayAnimation method can make a player play an animation immediately, while the StopAnimation method makes a player stop playing the animation triggered by PlayAnimation.
The PlayAnimation method has 3 parameters:
- Parameter 1: Animation to be played
- Parameter 2: You can set a callback function to trigger some logic when an animation has been played for a certain amount of time.
- This method supports Animation Events. You can set up an Animation Event to trigger a callback function that triggers desired logic when an animation has been played for a certain amount of time. For more information about how to set up an Animation Event, see Unity Animation Event.
- Note: The Animation Event can only trigger the callback function passed in. Therefore, the function name configured in the Animation Event does not take effect. All Animation Events will trigger the callback function. The callback function can read other parameters in the Animation Event.
- Parameter 3: You can set a mask for an animation to control the Avatar area affected by animation playback. For example, only the upper body plays the animation. For more information, see ParaAnimatorMask.
The following code example shows how PlayAnimation is used:
---@var Animation:UnityEngine.AnimationClip;
-- Play animation when click the gameobject
function PlayAnimation()
local me = ParaPlayerService.GetLocalPlayer();
-- Sync to all players in the room
self:SendMessageToAll("SyncAnimation", me.playerID);
end
-- Receive the message, and play animation on all players' clients
function SyncAnimation(playerID)
local player = ParaPlayerService.GetPlayerById(playerID);
player:PlayAnimation(Animation);
end
-- Stop playing animation when exit the collision
-- this will run on all clients
function OnPlayerTriggerExit(player)
player:StopAnimation();
end
Override an Animation
To dynamically replace an animation in the current animation controller in a World, use the ParaPlayer.SetOverrideAnimation method.
You need to specify the name of the animation to be overridden. If you set the animation to be overridden to nil, the original animation will be used.
The following code example uses this method to implement the scenario where a player starts dancing after walking into the Blue Hole dance floor:
function StartDance(playerID, normalIndex)
local player = CS.ParaPlayerService.GetPlayerById(playerID);
-- After entering the dance floor, the idle animation will be replaced by a dance animation.
player:SetOverrideAnimation("proxy_Idle", NormalDance);
end
function EndDance(playerID)
local player = CS.ParaPlayerService.GetPlayerById(playerID);
-- When leaving the dance floor, it will revert back to the normal idle animation.
player:SetOverrideAnimation("proxy_Idle", nil);
end
You can find the animation names in official animation controllers in the following directory: ParaAvatarSDK/ToolBox/ArtAssets/Animations/AnimationClips
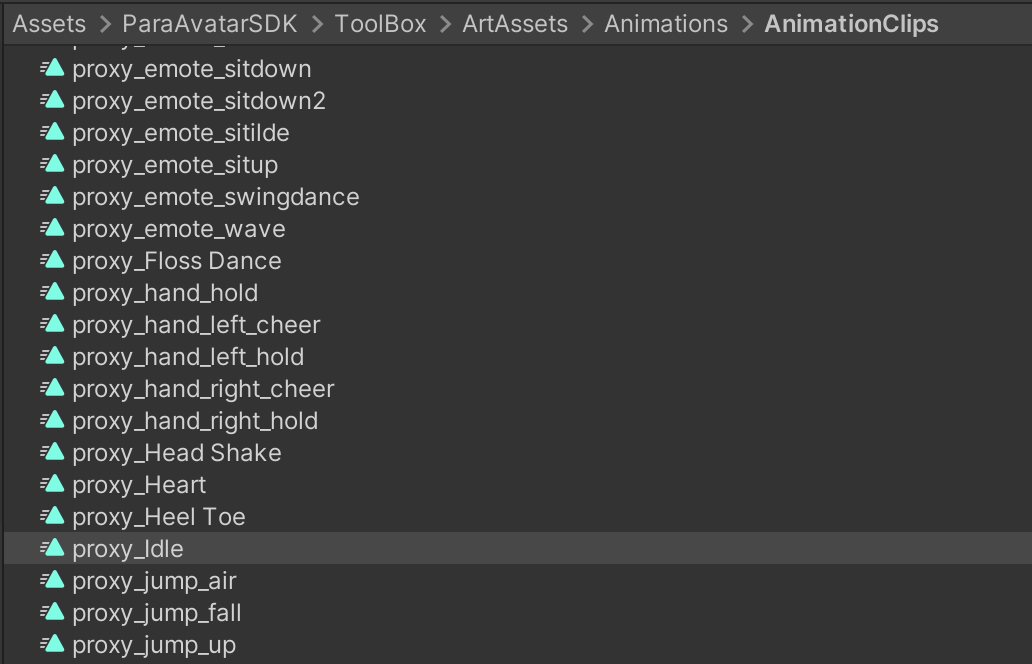
Override an Animation Controller
If you want players in your World to have completely different animation logic, you can override the current animation controller. This is also the most complex way to control animations. With this method, you can create your own brand new action game!
First, you need to configure a new animation controller that includes the correct state switching logic, and then use the ParaPlayer.SetOverrideAnimationController method to override the current animation controller on the player with the new animation controller. Later you can use relevant Unity APIs to control the new animation controller.
If you set the animation controller to be overridden to nil, the original animation controller will be used.
See also
About AnimatorOverrideController
In Unity, AnimatorOverrideController is a component used to override specific animations in an AnimatorController. It allows developers to change animations at runtime, enabling dynamic replacement and blending of animations.
Updated 2 days ago