Functions
Besides calling APIs to complete logic editing, you can also use custom functions to do that.
Functions are some blocks of code that can be executed and called repeatedly. They are usually used to finish up a fixed logic or used as callback.
Basic function Composition
A function is composed of the following:
- Scope: With local or without local. Whether "local" is included determines the scope for calling the function. Please see the details below.
- Keyword: function
- Function name
- Function parameters, which are placed within the brackets.
- Actual logic code block of the function
- Keyword: end
A function will be executed after being called. By doing this: You can call a function by writing its name and inputting parameters. If there is a return value for the function, you can define a variable to store the return value or call the funtion directly on where the return value needs to be used.
Here is an example for a simple "addition" function:
-- This function will add two parameters up and return the result.
local function addNumbers(num1, num2)
local result = num1 + num2
return result
end
print(addNumbers(1, 2)) -- It prints 3. You can call this function directly on where the return value is needed.
local seven = addNumbers(3, 4)
print(seven) -- It prints 7. You can also store the return value in a variable and use it later.
About the local keyword
If a function or a variable is defined as local, it is available only for the current script and can only be called by subsequent code. In other words, a local function written at the bottom of a file cannot be called at the beginning of the file, which will cause a call error.
If a functionor a variable is not defined as local, it can be called by any script or be called at any place in a file, no matter where it is written.
Call Methods
A function belongs to a class or table. When a function is a part of a class, it's called a method. Its first parameter usually indicates its self.
When you call a method, you can use a colon (:). This is the preferred way with straight-to-the-point writing, meaning that it automatically passes the first parameter (self) to the method.
Or, you can use a dot (.). However, this requires you to write out the passing of the parameter (self) as well. In other words, a colon (:) is the shorthand of a dot (.), in some sense.
Some of the C# APIs are static methods. You can use a dot (.) to call them. This type of method does not require passing the self as a parameter.
So...
If you're calling a static method, use a dot (.)
If you want to call a non-static method belonging to an instance, use a colon (:)
In the script, to call all ParaScript methods, you need to write: self:FunctionName()
For example: We can make the local player jump using a script. Here is the code.
local localPlayer = ParaPlayerService.GetLocalPlayer() -- Static method; use a dot (.) to call.
if localPlayer ~= nil then
localPlayer:Jump() -- Use a colon (:) to call. Here, Jump is the method of the localPlayer.
localPlayer.Jump(localPlayer) -- Use a dot (.) to call.
end)
end
Define Methods
Define a method in a table, with the method name being the key and method body being the value.
Meanwhile, you need to set the first parameter as self. This parameter (self) points to the table, and if you have other custom parameters, you need to list them after the self parameter.
When you use a colon (:) to call this method, you are automatically passing self as the first parameter to the method.
Below is a demonstration of how you define a method in a table. (It shows multiple ways of writing the code, please compare and use them as you see fit.)
-- Definition method 1:
local door = {
isOpen = true,
changeOpen = function(self, isOpen)
self.isOpen = isOpen
print(self.isOpen)
end
}
-- Definition method 2:
function door:changeOpen2(isOpen)
self.isOpen = isOpen
print(self.isOpen)
end
-- Definition method 3
function door.changeOpen3(isOpen)
-- Since it does not use a colon, the self is not automatically passed within the method
-- It cannot read the door content through the self inside the method
door.isOpen = isOpen
print(door.isOpen)
end
-- Comparing call methods
door:changeOpen(false) -- Recommended, output: false
door.changeOpen(self, false) -- Output: false
local aMethod = door.changOpen;
aMethod(door, false) -- Output: false
Callbacks
A callback is a function passed as an argument to another function.
Some commonly used functions use callbacks, which means treating a function as an argument of another function. You can use it in this way:
-- Delay 1 second before outputting Hello World
setTimeout(function()
print("Hello World")
end, 1000)
Call among scripts
When you need to call a function from another script, you need to obtain the corresponding script container ParaScript object first. Only then can you use the non-local function from its Lua script.
Shown below:
-- Declare a variable of ParaScript type, and drag the corresponding component and assign a value in the Unity Inspector
---@var paraScript:ParaScript
---@end
-- You can execute the following actions once you have the corresponding ParaScript
-- Execute ParaScript API
paraScript:SendMessageToOwner("Message") -- Send a custom message to the Owner
-- Assume that the called Lua script has the same content as the current script, the following function is available
function hello()
print("Hello World")
end
-- Get the associated Lua script before you call the function
paraScript.script.hello() -- Output: Hello World
How to obtain ParaScript
- As shown below:
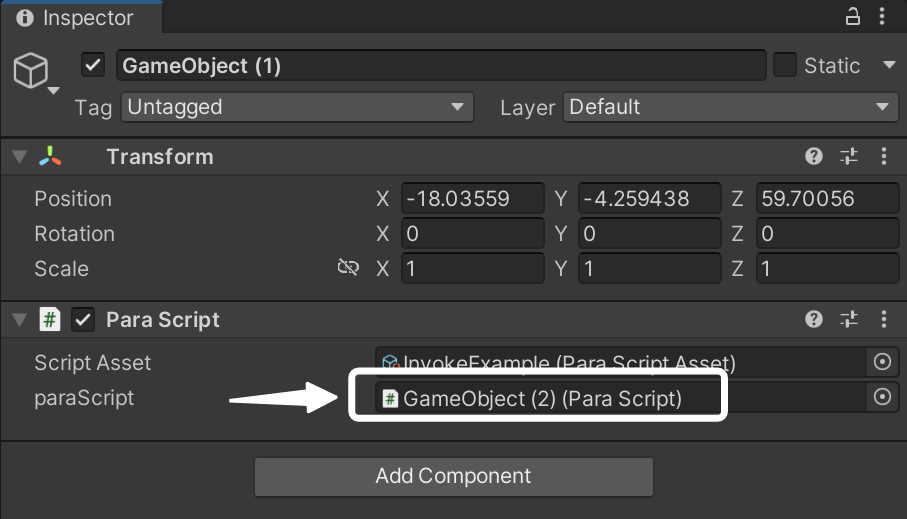
Declare a variable of ParaScript type, and drag the corresponding component and assign a value in the Unity Inspector.
Drag the corresponding component to the target location if two ParaScripts of the same GameObject want to refer to each another.
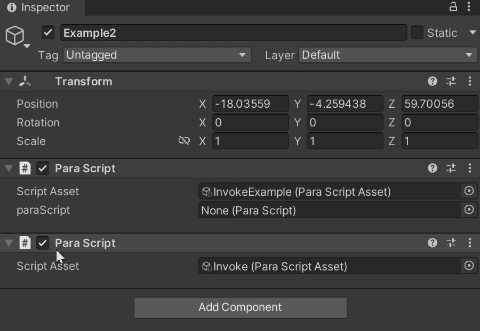
Associate the ParaScripts within the same GameObject.
- You can get other objects' ParaScript using the API provided by ParaScript:
- ParaScript GetParaScript(string name): Obtain ParaScript through the name. If multiple ParaScripts have the same name, return the first one by default.
- ParaScript[] GetParaScripts(string name): If multiple ParaScripts have the same name, you can obtain an array using this method.
Updated over 2 years ago