Declaration
public Action<PointerEventData> onBeginDrag;
Description
Assign callback methods to the property through Lua to obtain notifications when it gets dragged.
As shown in the figure below: 1. Create a UI->Panel, and suppose we name it as UIDragTest and put it under the Canvas. 2. Create another draggable image under Panel, and mount the ParaUIDragger component on the image's GameObject. 3. Click UIDragTest in the Hierarchy and drag the sample code below to the Inspector panel, and we will get the same result shown below.
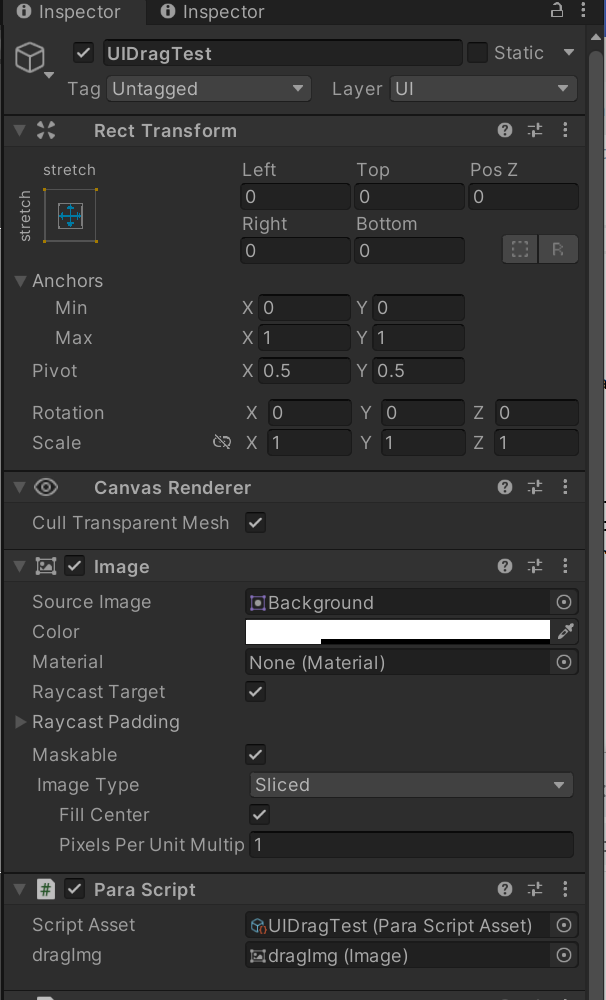
---@var dragImg : UnityEngine.UI.Image
---@end
-- UIDragTest.lua example code
local Vector3 = CS.UnityEngine.Vector3;
-- Start is called before the first frame update
function Start()
local dragger = dragImg:GetComponent(typeof(CS.ParaUIDragger));
local dragingGameObject = nil;
dragger.onBeginDrag = function(eventData)
print("drag begin position:",eventData.position);
dragingGameObject = CS.UnityEngine.GameObject.Instantiate(dragger.gameObject);
dragingGameObject.transform:SetParent(self.transform,false);
end
dragger.onDrag = function(eventData)
if dragingGameObject == nil then
return
end
-- draging change the image position
local vPos = dragingGameObject.transform.localPosition;
dragingGameObject.transform.localPosition = Vector3(vPos.x + eventData.delta.x,vPos.y + eventData.delta.y,0);
end
dragger.onEndDrag = function(eventData)
print("drag end, destroy the draging object");
CS.UnityEngine.GameObject.Destroy(dragingGameObject);
dragingGameObject = nil;
end
end